Xdebug is a PHP extension which enables you to debug your code, same as if you were using something like VisualStudio with C#. In order to install and enable Xdebug on Ubuntu, you just need to run the command:
sudo apt-get install php5-xdebug
After this package has been installed, you have to enable remote debugging on Xdebug (it is disabled by default). Add the line:
xdebug.remote_enable=on
to the /etc/php5/conf.d/xdebug.ini file. In order for the changes to take effect, you will have to restart Apache:
/etc/init.d/apache2 restart
If you ever want to verify what the Xdebug settings are set to, you can see them on the default
phpinfo()
page.Now that we've got Xdebug running, go ahead and create a new PHP project in NetBeans and name it 'PHPIncrement_Add':
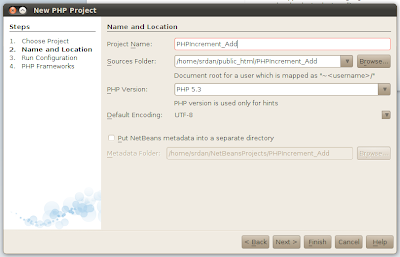
You can leave the 'Run Configuration' screen settings set to the defaults:
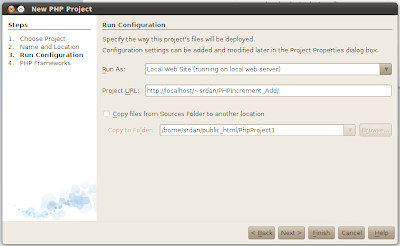
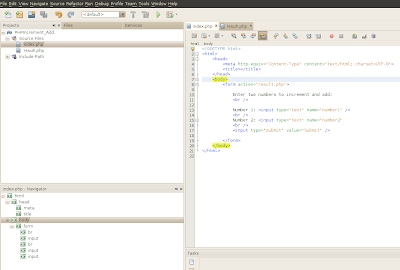
When results.php opens in the editor window, modify it to look like the following:
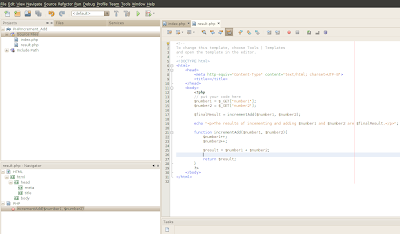
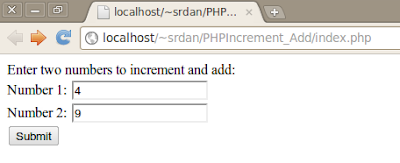
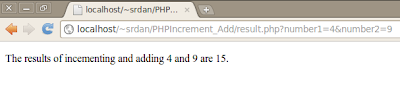
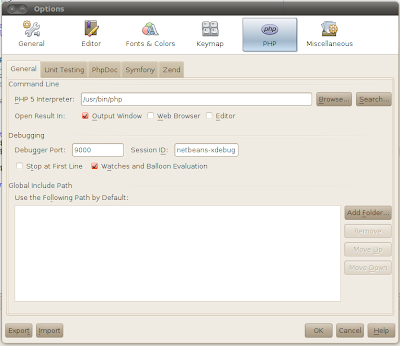
Next we can go ahead and set some breakpoints by clicking in the left hand margin of the editor window, where the line numbers are shown:
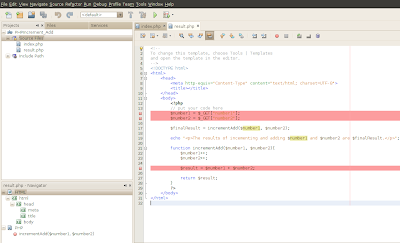
At the start we are presented with the index.php page, so just go ahead and enter some numbers and hit the 'Submit' button. Once this is done, you will automatically switch back to NetBeans, with the debugging session started:
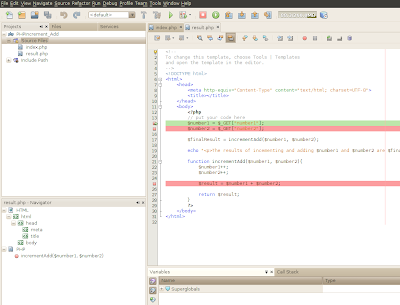
If you select the 'Variables' tab on the bottom pane and press the 'Step Into' (F7) button, you can see the values of $number1 and $number2:
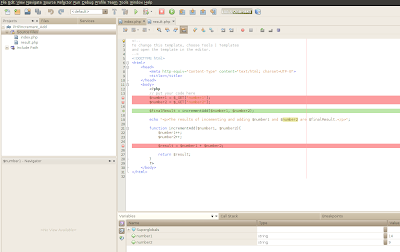
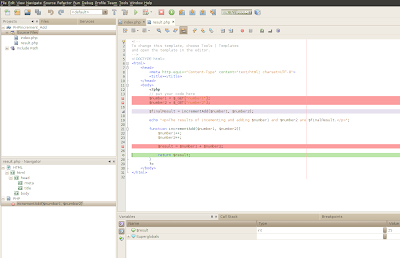
Hit the 'Continue' button and you will see the results.php page displaying the results of the calculation.
So, in this post we've successfully installed and configured Xdebug and got it working with our NetBeans IDE, as well creating a simple project and going through a basic debugging session. Please keep in mind that this is a very simple example and that debugging for such a basic project would probably not be necessary. The debugger is especially useful when we have a obscure bug which we need to track down, we want to see the flow of control in a complex piece of code or maybe verify what values are being passed to the database.
No comments:
Post a Comment